Maven安装
https://blog.csdn.net/tirster/article/details/123418269
数据库连接池 Druid(对于我要做的项目来说用不上,不是面向多用户的)
Druid 连接池介绍 - 简书 (jianshu.com)
MyBatis
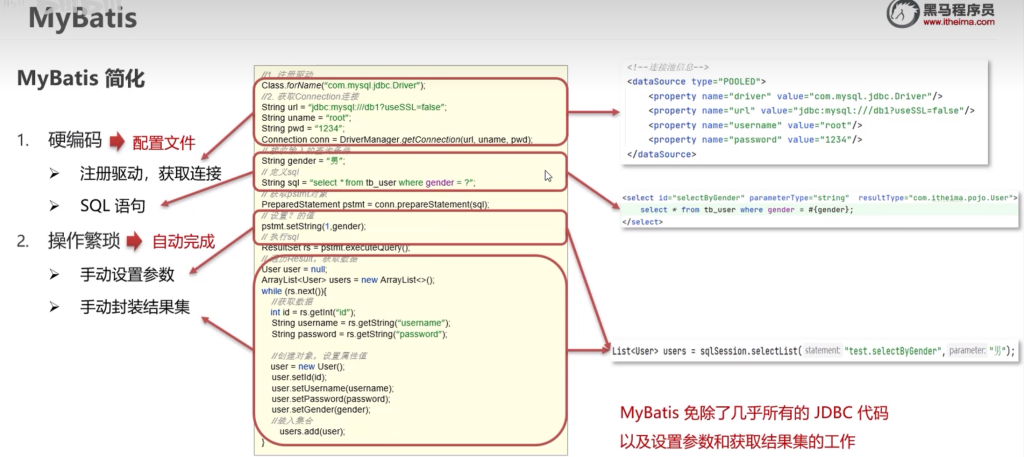
新建项目,设置依赖
在IntelliJ中新建个空项目,再新建maven框架的模块,取名mybatis_demo
在pom.xml中加入mybatis等依赖,日志依赖需要把logback.xml拖入main的资源目录
<dependencies>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.5</version>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.1.1</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>1.2.3</version>
</dependency>
</dependencies>
编写Mybatis核心配置文件
从 XML 中构建 SqlSessionFactory,在资源下新建mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="org.postgresql.Driver"/>
<property name="url" value="jdbc:postgresql://localhost:5432/Test0"/>
<property name="username" value="postgres"/>
<property name="password" value="~"/>
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="UserMapper.xml"/>
</mappers>
</configuration>
映射SQL
在资源文件夹里新建UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="test">
<select id="selectAll" resultType="dataReceiver.User">
select * from Test0;
</select>
</mapper>
同时在main里新建类dataReceiver.User
package dataReceiver;
public class User {
private int debt;
private String name;
public int getDebt() {
return debt;
}
public void setDebt(int debt) {
this.debt = debt;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "User{" +
"debt=" + debt +
", name='" + name + '\'' +
'}';
}
}
//Alt+Insert快捷构建函数
编写测试类
main里新建MyBatisDemo类
import dataReceiver.User;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.apache.ibatis.session.SqlSession;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class MybatisDemo {
public static void main(String[] args) throws IOException {
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//执行sql
List<User> users = sqlSession.selectList("test.selectAll");
System.out.println(users);
//释放资源
sqlSession.close();
}
}
报错:
严重: Connection error:
org.postgresql.util.PSQLException: 不支援 10 验证类型。请核对您已经组态 pg_hba.conf 文件包含客户端的IP位址或网路区段,以及驱动程序所支援的验证架构模式已被支援。
前往修改pg_hba.conf,重启postgreSql服务
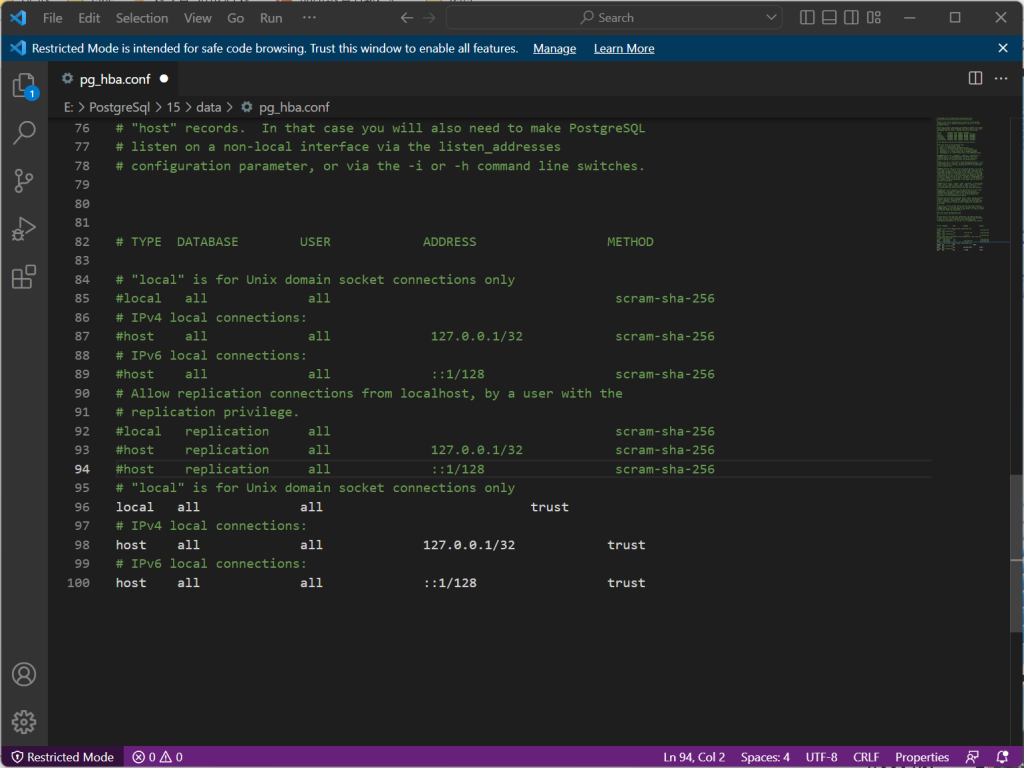
Mapper代理开发
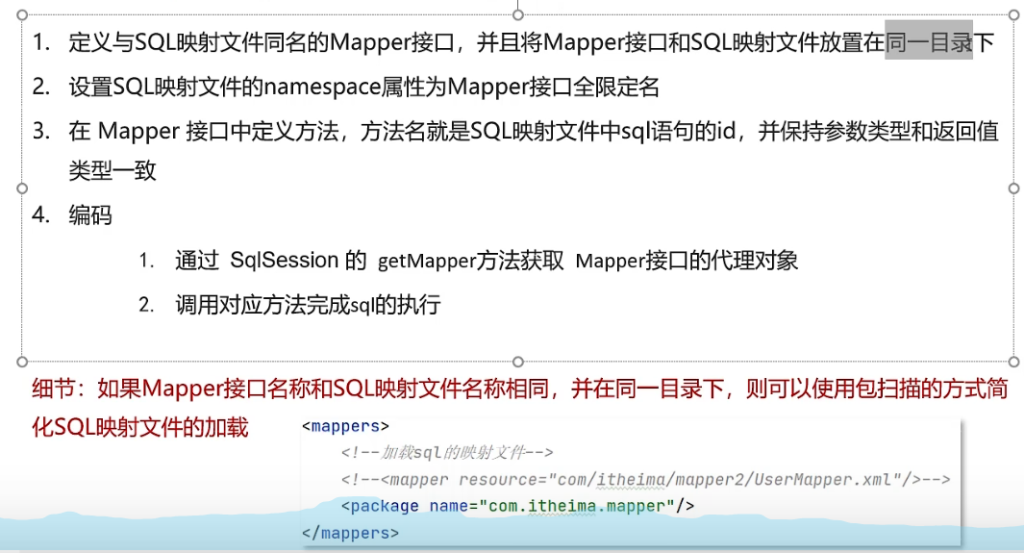
定义mapper
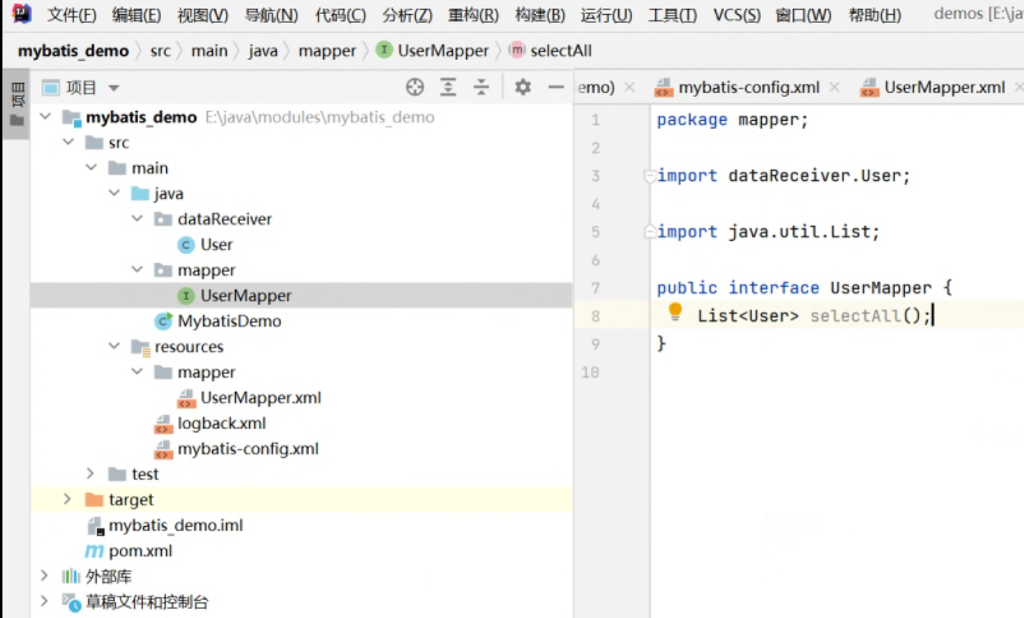
把mapper.xml置于同目录下,修改namespace
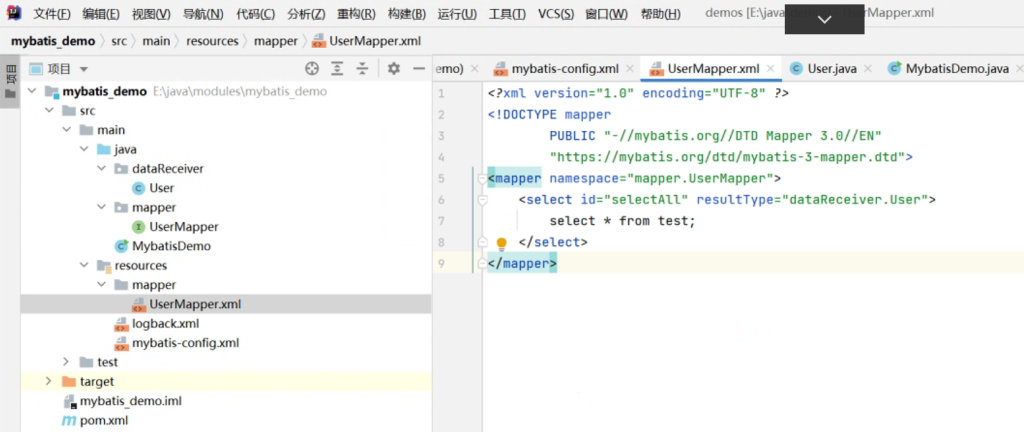
修改映射文件mapper.xml相对位置
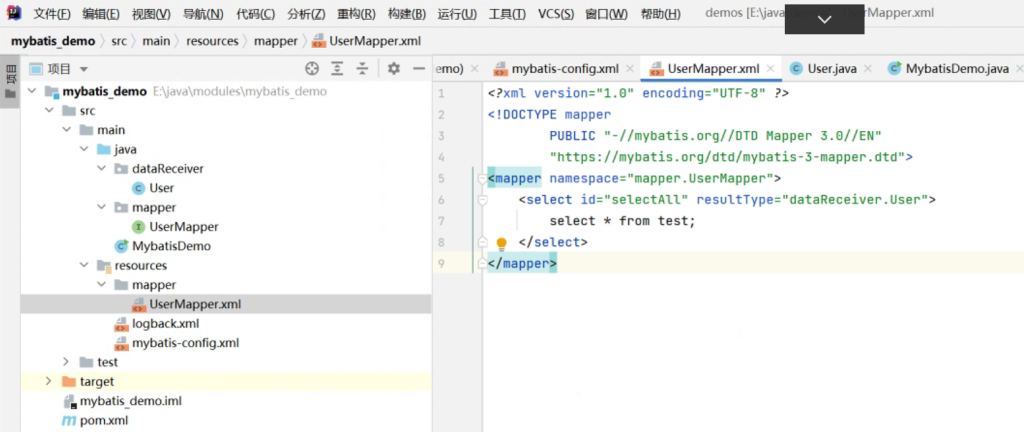
测试类
public class MybatisDemo2 {
public static void main(String[] args) throws IOException {
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//执行sql
//List<User> users = sqlSession.selectList("test.selectAll");
//获取对于UserMapper接口的代理对象
UserMapper userMapper = sqlSession.getMapper(UserMapper.class);
List<User> users = userMapper.selectAll();
System.out.println(users);
//释放资源
sqlSession.close();
}
}
包扫描,一次导入所有mapper类,需把所有mapper类放在同一文件夹下
<mappers>
<!--<mapper resource="mapper/UserMapper.xml"/>-->
<package name="mapper"/>
</mappers>
<typeAliases>别名
增查删改练习
创建示例表
-- 删除tb_brand表
drop table if exists tb_brand;
-- 创建tb_brand表
create table tb_brand
(
-- id 主键
id int primary key auto_increment,
-- 品牌名称
brand_name varchar(20),
-- 企业名称
company_name varchar(20),
-- 排序字段
ordered int,
-- 描述信息
description varchar(100),
-- 状态:0:禁用 1:启用
status int
);
-- 添加数据
insert into tb_brand (brand_name, company_name, ordered, description, status)
values ('三只松鼠', '三只松鼠股份有限公司', 5, '好吃不上火', 0),
('华为', '华为技术有限公司', 100, '华为致力于把数字世界带入每个人、每个家庭、每个组织,构建万物互联的智能世界', 1),
('小米', '小米科技有限公司', 50, 'are you ok', 1);
SELECT * FROM tb_brand;
新建数据类Brand
package dataReceiver;
/**
* 品牌
*
* alt + 鼠标左键:整列编辑
*
* 在实体类中,基本数据类型建议使用其对应的包装类型
*/
public class Brand {
// id 主键
private Integer id;
// 品牌名称
private String brandName;
// 企业名称
private String companyName;
// 排序字段
private Integer ordered;
// 描述信息
private String description;
// 状态:0:禁用 1:启用
private Integer status;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getBrandName() {
return brandName;
}
public void setBrandName(String brandName) {
this.brandName = brandName;
}
public String getCompanyName() {
return companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public Integer getOrdered() {
return ordered;
}
public void setOrdered(Integer ordered) {
this.ordered = ordered;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
@Override
public String toString() {
return "Brand{" +
"id=" + id +
", brandName='" + brandName + '\'' +
", companyName='" + companyName + '\'' +
", ordered=" + ordered +
", description='" + description + '\'' +
", status=" + status +
'}';
}
}
在Test里新建测试类
package test;
import dataReceiver.Brand;
import mapper.BrandMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import org.junit.Test;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class MyBatisTest {
@Test
public void testSelectAll() throws IOException {
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
List<Brand> brands = brandMapper.selectAll();
System.out.println(brands);
//释放资源
sqlSession.close();
}
}
BrandMapper类
package mapper;
import dataReceiver.Brand;
import java.util.List;
public interface BrandMapper {
public List<Brand> selectAll();
}
BrandMapper映射表
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.BrandMapper">
<select id="selectAll" resultType="dataReceiver.Brand">
select * from tb_brand;
</select>
</mapper>
数据库字段与数据接收类的字段名不一致时的解决方法:
1 sql查询时起别名
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.BrandMapper">
<!--<select id="selectAll" resultType="dataReceiver.Brand">
select * from tb_brand;
</select>-->
<select id="selectAll" resultType="dataReceiver.Brand">
select id,brand_name as BrandName,company_name as companyName,ordered,description,status from tb_brand;
</select>
</mapper>
将sql片段存储为别名方便调用
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.BrandMapper">
<!--<select id="selectAll" resultType="dataReceiver.Brand">
select * from tb_brand;
</select>-->
<sql id="brand_column">
id,brand_name as BrandName,company_name as companyName,ordered,description,status
</sql>
<select id="selectAll" resultType="dataReceiver.Brand">
select <include refid="brand_column"/> from tb_brand;
</select>
</mapper>
2 resultmap
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"https://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.BrandMapper">
//id唯一标识,type映射的类型,id(主键),result(普通字段),column数据库字段名,property别名
<resultMap id="brandResultMap" type="dataReceiver.Brand">
<result column="brand_name" property="brandName" />
<result column="company_name" property="companyName" />
</resultMap>
<select id="selectAll" resultMap="brandResultMap">
select * from tb_brand;
</select>
</mapper>
查询时接收参数
//BrandMapper.xml
<select id="selectById" resultMap="brandResultMap">
//#{}替换为?防止注入
//${}拼sql,存在被注入,可用于动态表名
select * from tb_brand where id = #{id};
</select>
//可以设置传入变量类型
<select id="selectById" parameterType="int" resultMap="brandResultMap">
//BrandMapper
//public可以不写
Brand selectById(int id);
//MyBatisTest
@Test
public void testSelectById() throws IOException {
//接受参数
int id = 1;
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
Brand brand = brandMapper.selectById(1);
System.out.println(brand);
//释放资源
sqlSession.close();
}
特殊字符处理
xml中 <号无法直接使用
1 转义字符 <
2 CDATA区
<select id="selectById" parameterType="int" resultMap="brandResultMap">
select * from tb_brand where id
<![CDATA[
<
]]>
#{id};
</select>
条件查询
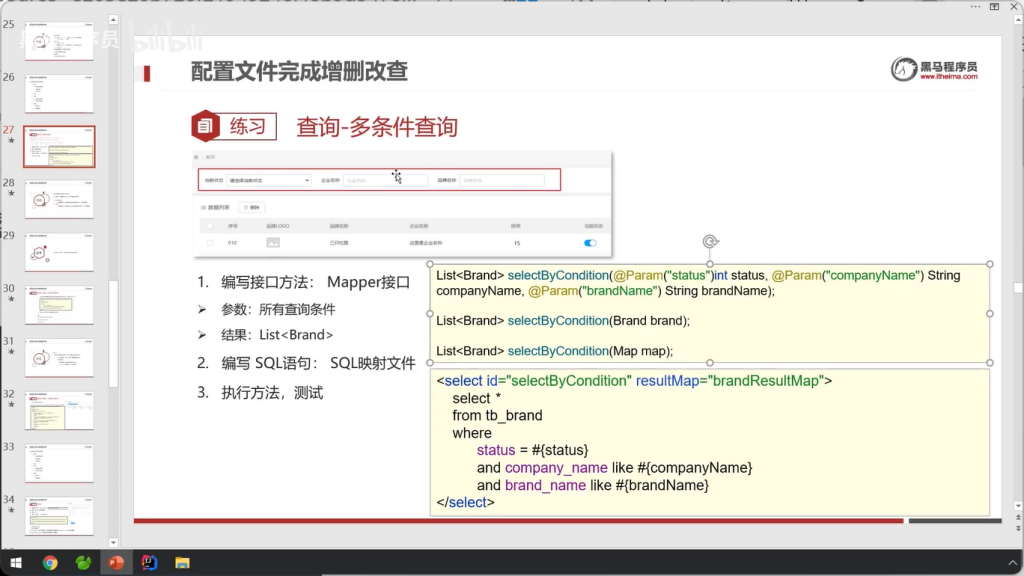
方法1 函数设置注解Param
//BrandMapper.xml
<!--条件查询-->
<select id="selectByCondition" resultMap="brandResultMap">
select * from tb_brand where
status = #{status}
and company_name like #{companyName}
and brand_name like #{brandName}
</select>
//BrandMapper
List<Brand> selectByCondition(@Param("status") int status,
@Param("companyName") String companyName,
@Param("brandName") String brandName);
//MyBatisTest
@Test
public void testSelectByCondition() throws IOException {
//接受参数
int status = 1;
String companyName = "华为";
String brandName = "华为";
companyName = "%" + companyName + "%";
brandName = "%" +brandName + "%";
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
List<Brand> brands = brandMapper.selectByCondition(status,companyName,brandName);
System.out.println(brands);
//释放资源
sqlSession.close();
}
方法2 传入对象
//BrandMapper
List<Brand> selectByCondition(Brand brand);
//MyBatisTest
@Test
public void testSelectByCondition() throws IOException {
//接受参数
int status = 1;
String companyName = "华为";
String brandName = "华为";
companyName = "%" + companyName + "%";
brandName = "%" +brandName + "%";
//封装对象
Brand brand = new Brand();
brand.setStatus(status);
brand.setCompanyName(companyName);
brand.setBrandName(brandName);
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
List<Brand> brands = brandMapper.selectByCondition(brand);
System.out.println(brands);
//释放资源
sqlSession.close();
}
3 使用map集合
//BrandMapper
List<Brand> selectByCondition(Map brand);
//MyBatisTest
@Test
public void testSelectByCondition() throws IOException {
//接受参数
int status = 1;
String companyName = "华为";
String brandName = "华为";
companyName = "%" + companyName + "%";
brandName = "%" +brandName + "%";
/* //封装对象
Brand brand = new Brand();
brand.setStatus(status);
brand.setCompanyName(companyName);
brand.setBrandName(brandName);*/
Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
List<Brand> brands = brandMapper.selectByCondition(map);
System.out.println(brands);
//释放资源
sqlSession.close();
}
动态条件查询
多条件动态查询
//BrandMapper.xml
<!-- 动态条件查询-->
<select id="selectByCondition" resultMap="brandResultMap">
select * from tb_brand where
<if test="status != null">
status = #{status}
</if>
<if test="companyName != null and companyName != '' ">
and company_name like #{companyName}
</if>
<if test="brandName != null and brandName != '' ">
and brand_name like #{brandName}
</if>
</select>
//MyBatisTest
@Test
public void testSelectByCondition() throws IOException {
//接受参数
int status = 1;
String companyName = "";
String brandName = "";
companyName = "%" + companyName + "%";
brandName = "%" +brandName + "%";
/* //封装对象
Brand brand = new Brand();
brand.setStatus(status);
brand.setCompanyName(companyName);
brand.setBrandName(brandName);*/
Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
List<Brand> brands = brandMapper.selectByCondition(map);
System.out.println(brands);
//释放资源
sqlSession.close();
}
问题:当status不存在而后者条件存在时会导致sql语句多了and从而报错
解决方法:
1 在where后添加1=1的恒等式
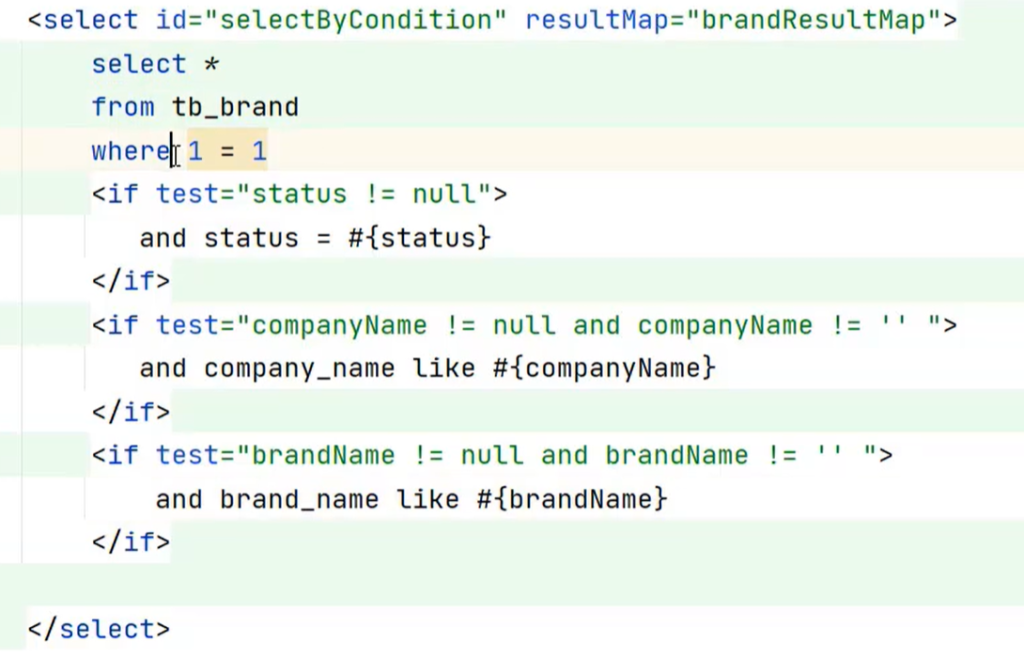
2<where> 替代 where
<!--条件查询-->
<select id="selectByCondition" resultMap="brandResultMap">
select * from tb_brand
<where>
<if test="status != null">
status = #{status}
</if>
<if test="companyName != null and companyName != '' ">
and company_name like #{companyName}
</if>
<if test="brandName != null and brandName != '' ">
and brand_name like #{brandName}
</if>
</where>
</select>
单条件动态查询
//BrandMapper.xml
<select id="selectByConditionSingle" resultMap="brandResultMap">
select *
from tb_brand
<where>
<choose>
<when test="status !=null">
status = #{status}
</when>
<when test="companyName != null and companyName != '' ">
company_name like #{companyName}
</when>
<when test="brandName != null and brandName != '' ">
brand_name like #{brandName}
</when>
</choose>
</where>
</select>
//MyBatisTest.java
@Test
public void testSelectByConditionSingle() throws IOException {
//接受参数
int status = 1;
String companyName = "小米";
String brandName = "";
companyName = "%" + companyName + "%";
brandName = "%" +brandName + "%";
//封装对象
Brand brand = new Brand();
brand.setStatus(status);
//brand.setCompanyName(companyName);
//brand.setBrandName(brandName);
/* Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);*/
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
List<Brand> brands = brandMapper.selectByConditionSingle(brand);
System.out.println(brands);
//释放资源
sqlSession.close();
}
添加
一直在疑惑为什么我的IDE不能自动给sql补全,原来要按住alt+enter选择语言诸如设置为postgresql
注意是否设置自动提交事务
//brandMapper.java
//添加
void add(Brand brand);
//brandMapper.xml
<insert id="add">
insert into tb_brand (brand_name, company_name, ordered, description, status)
values (#{brandName},#{companyName},#{ordered},#{description},#{status});
</insert>
//MyBatisTest.java
@Test
public void testAdd() throws IOException {
//接受参数
int status = 1;
String companyName = "阿巴阿巴";
String brandName = "阿巴";
String description = "hahah";
int order = 100;
//封装对象
Brand brand = new Brand();
brand.setStatus(status);
brand.setCompanyName(companyName);
brand.setBrandName(brandName);
brand.setOrdered(order);
brand.setDescription(description);
/* Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);*/
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//自动提交事务
//SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
brandMapper.add(brand);
//提交事务
sqlSession.commit();
//释放资源
sqlSession.close();
}
添加时获取主键
//brandMapper.xml
<insert id="add" useGeneratedKeys="true" keyProperty="id">
insert into tb_brand (brand_name, company_name, ordered, description, status)
values (#{brandName},#{companyName},#{ordered},#{description},#{status});
</insert>
//MyBatisTest.java
@Test
public void testAdd() throws IOException {
//接受参数
int status = 1;
String companyName = "阿巴阿巴";
String brandName = "阿巴";
String description = "hahah";
int order = 100;
//封装对象
Brand brand = new Brand();
brand.setStatus(status);
brand.setCompanyName(companyName);
brand.setBrandName(brandName);
brand.setOrdered(order);
brand.setDescription(description);
/* Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);*/
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//自动提交事务
//SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
brandMapper.add(brand);
int id = brand.getId();
System.out.println(id);
//提交事务
sqlSession.commit();
//释放资源
sqlSession.close();
}
修改
修改全部字段
//brandMapper.java
int update(Brand brand);
//brandMapper.xml
<update id="update">
update tb_brand
set brand_name = #{brandName},
company_name = #{companyName},
ordered = #{ordered},
description = #{description},
status = #{status}
where id = #{id};
</update>
//MyBatisTest.java
@Test
public void testUpdate() throws IOException {
//接受参数
int status = 1;
String companyName = "阿巴阿巴111";
String brandName = "阿巴";
String description = "hahah";
int order = 200;
int id = 7;
//封装对象
Brand brand = new Brand();
brand.setStatus(status);
brand.setCompanyName(companyName);
brand.setBrandName(brandName);
brand.setOrdered(order);
brand.setDescription(description);
brand.setId(id);
/* Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);*/
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//自动提交事务
//SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
int count = brandMapper.update(brand);
System.out.println(count);
//提交事务
sqlSession.commit();
//释放资源
sqlSession.close();
}
动态字段修改
//brandMapper.xml
<update id="update">
update tb_brand
<set>
<if test="brandName!=null and brandName!=''">
brand_name = #{brandName},
</if>
<if test="companyName!=null and companyName!=''">
company_name = #{companyName},
</if>
<if test="ordered!=null">
ordered = #{ordered},
</if>
<if test="description != null and description != ''">
description = #{description},
</if>
<if test="status != null">
status = #{status}
</if>
</set>
where id = #{id};
</update>
//myBatisTest
@Test
public void testUpdate() throws IOException {
//接受参数
int status = 0;
String companyName = "阿巴阿巴111";
String brandName = "阿巴";
String description = "hahah";
int order = 200;
int id = 7;
//封装对象
Brand brand = new Brand();
brand.setStatus(status);
/* brand.setCompanyName(companyName);
brand.setBrandName(brandName);
brand.setOrdered(order);
brand.setDescription(description);*/
brand.setId(id);
/* Map map = new HashMap();
map.put("status",status);
map.put("companyName",companyName);
map.put("brandName",brandName);*/
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//自动提交事务
//SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
int count = brandMapper.update(brand);
System.out.println(count);
//提交事务
sqlSession.commit();
//释放资源
sqlSession.close();
}
删除
单个删除
//brandMapper.java
void deleteById(int id);
//brandMapper.xml
<delete id="deleteById">
delete from tb_brand where id = #{id};
</delete>
//myBatisTest
@Test
public void testDeleteById() throws IOException {
//接受参数
int id = 7;
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//自动提交事务
//SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
brandMapper.deleteById(id);
//提交事务
sqlSession.commit();
//释放资源
sqlSession.close();
}
多个删除
//brandMapper.java
void deleteByIds(@Param("ids") int[] ids);
//brandMapper.xml
<!--mybatis会将数组参数封装为一个Map集合
默认array=数组
可以用@Param注释改变map集合的默认key的名称
-->
<delete id="deleteByIds">
delete from tb_brand where id
in
<foreach collection="ids" item="id" separator="," open="(" close=")">
#{id}
</foreach>
;
</delete>
//myBatisTest
@Test
public void testDeleteByIds() throws IOException {
//接受参数
int[] ids = {11,12};
//加载mybtis核心配置文件,获取sqlSessionFactory
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
//获取sqlSession对象,用它执行sql
SqlSession sqlSession = sqlSessionFactory.openSession();
//自动提交事务
//SqlSession sqlSession = sqlSessionFactory.openSession(true);
//获取Mapper接口的代理对象
BrandMapper brandMapper = sqlSession.getMapper(BrandMapper.class);
//执行方法
brandMapper.deleteByIds(ids);
//提交事务
sqlSession.commit();
//释放资源
sqlSession.close();
}
注解开发
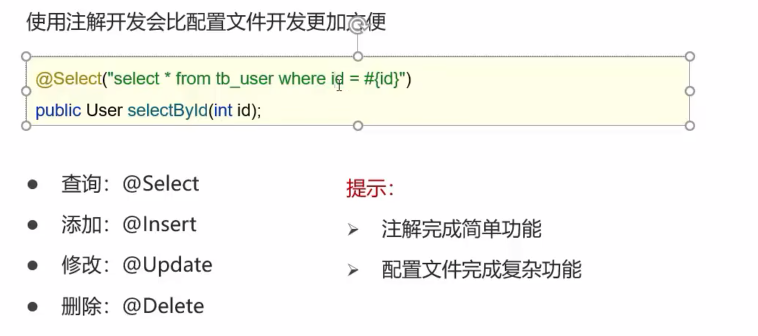
Comments NOTHING